Getting Started with ShellFolder
Requirements:
CBFS Shell
Contents
Introduction
The ShellFolder component allows your application to extend File Explorer, giving you capability to create a custom folder with items, custom context menus, actions, and icons. Users can navigate to the folders that you create and interact with the content just like any other folder on the system. You are in full control of defining the items, item content, and menu items that users will see.
Each instance of the component is capable of managing a custom region of files and folders under File Explorer. Before continuing, it is recommended to download CBFS Shell in order to follow along with this tutorial.
Creating a Folder
Set your preferred folder settings to begin. At a minimum one should set the DisplayName and Location properties. For a full list of available properties please see our Online Documentation.
import callback.CBFSShell;
ShellFolder m_Folder = new ShellFolder();
m_Folder.DisplayName = "Sales Department";
m_Folder.Location = Constants.NS_LOCATION_DESKTOP;
The next step is to call Install. This will load a DLL into File Explorer to facilitate communication with the Windows Shell. Installation should be done at least once before using the library on a new system, but may be done several times to update the desired settings.
m_Folder.Install();
Installation will make the root folder visible in File Explorer, but it will not be ready to handle user interaction.
After that you should call the Initialize method to perform setup tasks such as preparing the DLL. Initialization should be done during your application session and only needs to be done once.
m_Folder.Initialize();
The Activate method starts the component. This action will make the virtual folder visible in File Explorer and allow users to interact with the folder. After the component has been Activated, events will fire that need to be handled.
m_Folder.Activate();
The ShellFolder component will ask for information to carry out the tasks of extending the Windows Shell. Handling the events allows you to populate your folder with items, metadata, and behavior.
Items
When the Shell asks for items, the ListItems event will fire, and items can be returned to the view by calling ListItem method inside this event. The ParentId parameter will contain an identifier of the folder whose contents should be retrieved.
private void OnListItems(object sender, ShellFolderListItemsEventArgs e) {
// Add a file item
var itemId = "0"; // Use your own logic to identify, name, and describe the item
var path = ""; // Set this to a path of a real file if desired
var name = "MyItem.txt";
var size = 1000;
var iconPath = "";
var iconIndex = 0;
var attributes = Constants.SFGAO_HASPROPSHEET | Constants.SFGAO_STORAGE | Constants.SFGAO_STREAM;
m_Folder.ListItem(e.TaskId, itemId, attributes, size, name, path, iconPath, iconIndex);
// Add a folder item
itemId = "1";
name = "MyFolder";
attributes = Constants.SFGAO_FOLDER | Constants.SFGAO_HASPROPSHEET | Constants.SFGAO_STORAGE | Constants.SFGAO_STREAM;
m_Folder.ListItem(e.TaskId, itemId, attributes, size, name, path, iconPath, iconIndex);
}
After the ListItems event returns, the folder will be populated with the listed items and they will be accessible within File Explorer.
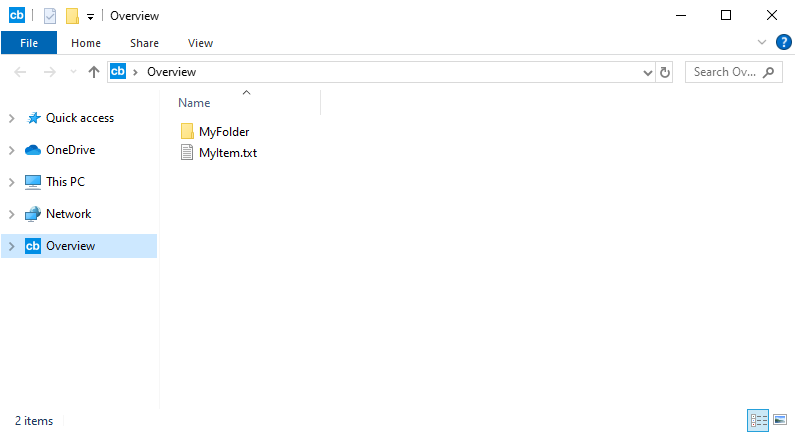
Properties
The GetProperties event will fire for each folder and non-folder item that is presented to the end-user. To add metadata, call AddProperty for each property associated with the item. These properties will be displayed in the Properties dialog box of File Explorer. It is only necessary to provide one of numericValue, dateValue, or stringValue parameters.
private void OnGetProperties(object sender, ShellFolderGetPropertiesEventArgs e) {
var propertyId = 4; // An identifier for System.Author defined by MSFT
var formatGUID = "{F29F85E0-4FF9-1068-AB91-08002B27B3D9}";
var propertyType = Constants.PROP_TYPE_STRING;
var numericValue = 0; // Set only for numeric properties
var dateValue = DateTime.MinValue; // Set only for date properties
var stringValue = "John Doe"; // Set only for string properties
// Add an Author property to the item
m_Folder.AddProperty(e.TaskId, formatGUID, propertyId, propertyType, numericValue, dateValue, stringValue);
}
Note: Please see Microsoft's Predefined Properties for a list of propertyId and formatGUID values.
Columns
The GetColumns event will fire to indicate that columns have been requested. To add columns to the view call the AddColumn method. The application should add a column for each custom property. Columns will be displayed in the view with the values associated with each item in GetProperties.
private void OnGetColumns(object sender, ShellFolderGetColumnsEventArgs e) {
var propertyID = 4; // System.Author
var formatGUID = "{F29F85E0-4FF9-1068-AB91-08002B27B3D9}";
var flags = Constants.SHCOLSTATE_ONBYDEFAULT;
var name = ""; // Set only if using a dynamic column
var defaultWidth = 0; // Set only if using a dynamic column
var viewFlags = 0; // Set only if using a dynamic column
// Add an Author column to the folder
m_Folder.AddColumn(e.TaskId, formatGUID, propertyID, flags, name, defaultWidth, viewFlags);
}
After the AddColumn method is called, the property values that correspond to the newly added column will be visible in File Explorer.
Note: If you would like to provide a column for a custom property separate from the list of registered properties provided by Windows, this is possible using dynamic columns. Please see the Online Documentation for more information.
Menus
The MergeContextMenu event will fire when the Shell is requesting a context menu. The application should add items to the menu using the AddMenuItem method. Menu items are usually associated with a Verb which represents a unique action. When you double-click a folder (or any item for that matter), the Shell builds a context menu and executes the default context menu item (the one in bold). Specify the IsDefault parameter if your item should be marked as the default item.
private void OnMergeMenu(object sender, ShellFolderMergeMenuEventArgs e) {
// Add a context menu item
m_Folder.AddMenuItem(e.TaskId, "OpenWithMyTool", "", "Open with my tool", true, true);
}
After AddMenuItem is called, the added menu item will be visible in the File Explorer's right-click context menu for any of the items within the virtual folder.
The InvokeMenu event will fire when a context menu item is executed. The Verb parameter will identify the unique action that should be performed. This allows you to insert the desired logic when an item is double-clicked or when a context menu item is clicked.
private void OnInvokeMenu(object sender, ShellFolderInvokeMenuEventArgs e) {
if (e.Verb == "OpenWithMyTool") {
Process.Start("MyApplication.exe")
}
}
Deactivation and Uninstall
To stop the virtual folder, call the Deactivate method. Deactivation will stop the processing requests from the Windows Shell and the virtual folder will be set to inactive state and may be activated again at a later time if desired.
m_Folder.Deactivate();
To remove the virtual folder, go ahead and call Uninstall. This action will unregister the DLL from the system, and is typically be performed when your application should be removed from the system.
m_Folder.Uninstall();
We appreciate your feedback. If you have any questions, comments, or suggestions about this article please contact our support team at support@callback.com.